Crafting Frontend: React — Update the Parent's State
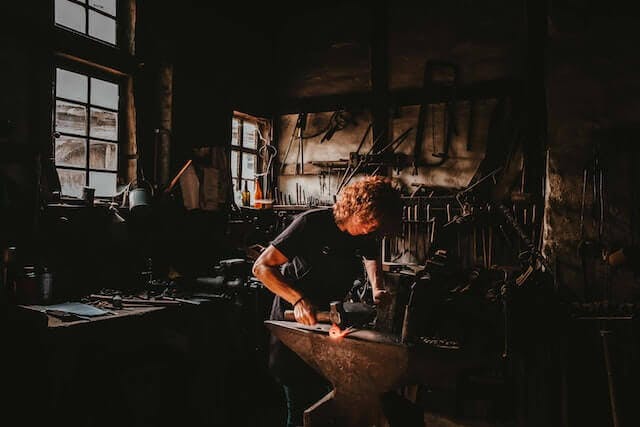
This post is part of the series Crafting Frontend
, the React version.
This will be a very simple post about one of the fundamental concepts in React. Using and updating the state from a parent component in React is pretty straightforward. To use a simple example, we need to first create the parent's state.
const Parent = () => {
const [state, setState] = useState(0);
return null;
};
Now let's just render the state in the UI:
const Parent = () => {
const [state, setState] = useState(0);
return <div>{state}</div>;
};
If we need to update from the child component, it should receive the state setter as a prop and use it accordingly.
import { useState } from 'react';
const Child = ({ updateState }) => (
<button onClick={() => updateState((state) => state + 1)}>+</button>
);
const Parent = () => {
const [state, setState] = useState(0);
return (
<div>
{state}
<Child updateState={setState} />
</div>
);
};
export default Parent;
This is just a simple example of rendering a counter and using a child component to update the counter. The parent component should pass the setter to the child and let it update the state for the parent to render the new updated value.
One of the problems that start to emerge is the infamous prop drilling. We can use the Context API to “transport” data, logic, and setter functions to any component that's wrapped in the context provider.
Or we start to think about using a state management tool such as mobx, jotai, redux, recoil, etc. Apparently, we have quite a lot of options out there.
Crafting Frontend
is a series of posts and experiments I'm doing to craft the art of frontend engineering. To see all the experiments I've been doing, follow the Crafting Frontend github repo.